Read, Write, Analyse & Refine Programs (Edexcel GCSE Computer Science)
Revision Note
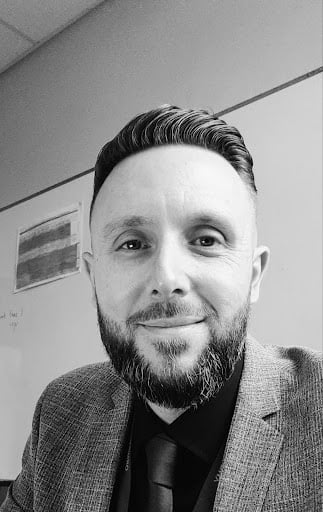
Author
Robert HamptonExpertise
Computer Science Content Creator
Read, Write, Analyse & Refine Programs
With the exception of the final question, which requires students to design and write a program from scratch, questions on paper 2 require students to analyse and refine given code by:
Correcting errors
Adding or rearranging lines
Selecting the correct line
Improving readability
Correcting errors
Find and correct the errors in this program
Python code - contain errors |
---|
# ----------------------------------------------------------------------- # This program calculates the area of a rectangle # -----------------------------------------------------------------------
|
This program contain 4 errors:
Syntax error - typo in variable name
lenght
(should belength
)Incorrect use of misspelled variable
lenght
in area calculationMissing bracket in first
print
statementIncorrect indentation for the
if
statement
Python code - corrected errors |
---|
# ----------------------------------------------------------------------- # This program calculates the area of a rectangle # -----------------------------------------------------------------------
|
Adding or rearranging lines
Add lines to the
calculate_grade()
function to assign letter grades based on the score:90 or above = A
80 - 89 = B
70 - 79 = C
Below 70 = F ("Fail")
Python code - incomplete |
---|
# ----------------------------------------------------------------------- # This function takes a score as input and returns the corresponding letter grade # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # This function gets user input for a score and calls the calculate_grade function # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # Main program # -----------------------------------------------------------------------
|
Python code - Complete |
# ----------------------------------------------------------------------- # This function takes a score as input and returns the corresponding letter grade # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # This function gets user input for a score and calls the calculate_grade function # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # Main program # -----------------------------------------------------------------------
|
Improving readability
Improve the readability of the following program by adding:
Layout
White space
Comments
Indentation
Python code - poor readability |
---|
|
Python code - good readability |
# ----------------------------------------------------------------------- """ This function calculates the total bill amount including VAT and optional tip Variables: bill_amount (float): The original bill amount. VAT(float): The tax rate (e.g., 0.20 for 20%). tip_percent (float, optional): The tip percentage (e.g., 15 for 15%). Defaults to 0. Returns: float: The total bill amount including VAT and tip. """ # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # Get user input # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # Ask about tip and calculate total # -----------------------------------------------------------------------
# ----------------------------------------------------------------------- # Print the final bill total # -----------------------------------------------------------------------
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?