Fundamental Components of Programs (Edexcel GCSE Computer Science)
Revision Note
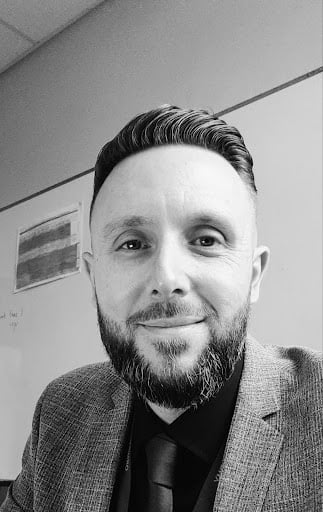
Author
Robert HamptonExpertise
Computer Science Content Creator
Constants, Variables, Initialisation & Assignment
Constants
A constant is an identifier set once in the lifetime of a program
Constants are named in all uppercase characters
Constants aid the readability and maintainability
If a constant needs to be changed, it should only need to be altered in one place in the whole program
Python examples |
---|
|
|
|
Variables
A variable is an identifier that can change in the lifetime of a program
Variables are named in lowercase characters unless combining multiple words
A variable can be associated a datatype when it is declared
When a variable is declared, memory is allocated based on the data type indicated
Python examples |
---|
|
|
|
Initialisation & assignment
A variable can be initialised with a value
The assignment operator
=
is used to set or change the value of a variable
Python examples |
---|
|
|
|
Inputs & Outputs
Inputs
The
input()
function is used to capture an input from the userAll inputs default to strings and may need converting to the required data type in the program
Python examples |
---|
|
|
|
Outputs
The
print()
function is used to output to the screen
Python examples |
---|
|
|
|
An output can be formatted to the users needs using string formatting
{}
Python examples |
---|
|
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?