Sequence & Selection (Edexcel GCSE Computer Science)
Revision Note
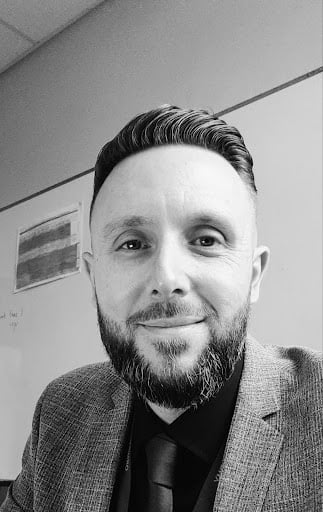
Author
Robert HamptonExpertise
Computer Science Content Creator
Sequence
What is sequence?
Sequence is a set of instructions executed one after another
Python example |
---|
""" This function calculates the area of a rectangle Inputs: length: The length of the rectangle width: The width of the rectangle Returns: The area of the rectangle """
# Calculate area
# ------------------------------------------------------------------------# Main program # ------------------------------------------------------------------------
|
In the example, the sequence of instructions is wrong and would cause a runtime error
In the
calculate_area()
function a value is returned before it is assignedThe correct sequence is:
# Calculate areaarea = length * width
return area
Selection
What is selection?
Selection is a programming construct that allows a program to execute in different ways based on the outcome of a condition
Selection is implemented using the
if
functionNested selection can be used, the use of '
else
' and 'elif
' is preferable for efficiency and to make logic clearer
Python examples |
---|
|
|
Nested selection
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?