Decisions in Computational Thinking (OCR A Level Computer Science)
Revision Note
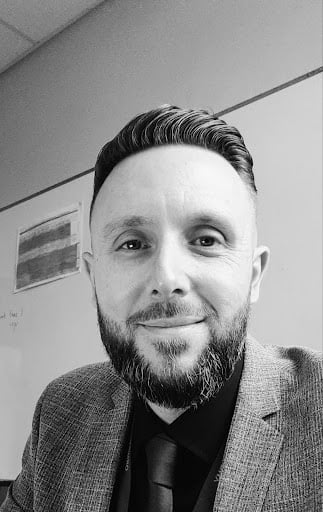
Author
Robert HamptonExpertise
Computer Science Content Creator
Identify the Points in a Solution where a Decision has to be Taken
Most languages use structured programming techniques including:
Sequence: one statement after another
Selection: Decision making, if/then/else, switch/case
Iteration: Loops, for/while/do while/do until
The purpose of these techniques is to aid the readability, understanding and maintainability of code
Python is an example of a block-structured language, which uses only these three constructs to control the flow of execution and data in a program. Each block of code should have a single entry and exit point and ideally minimise breaking out of iterative blocks. This is to prevent unintended consequences relating to the flow of control of a program such as entering or leaving a subroutine early or branching unintentionally
When designing algorithms, it is always best to plan the algorithm using flowcharts, pseudocode or structured english before coding in a language
Languages have specific syntax, constructs and idiosyncrasies that differ between other languages. This makes it challenging to create a solution that can immediately be implemented in another language
When determining the points in a solution where decisions are made, flowcharts are useful as they visually show the flow of control in a program. Decisions are clear and easy to follow, however flowcharts are time consuming to create
Pseudocode more accurately mimics the constructs of programming languages without the problem of syntax. As pseudocode has no syntax, any way of expressing an algorithm is acceptable as long as the pseudocode meaning is clear
Structured English can be an alternative to pseudocode but is usually more verbose and imprecise
How do you Identify the Points in a Solution where a Decision has to be Taken?
Most errors in a program occur when evaluating a Boolean condition, whether in a sequence as part of a statement, iteration or selection. It is therefore important to be careful when creating Boolean conditions, especially long, complex conditions involving multiple clauses
Decisions in programs usually occur in two situations, an if statement/select case or a loop, usually a while loop
Selection, also known as branching, involves directing the flow of control of a program, dependent on a Boolean condition or set of conditions
Iteration involves repeating a sequence of instructions based on a stopping Boolean condition
Determine how Decisions Affect Flow through a Program
Decisions affect the flow of control of a program
Decisions are Boolean conditions encountered in selection structures such as if/then/else statements and iteration structures such as while loops
If statements and switch/case statements can consist of many decision points as illustrated below. Each decision point directs the program through different statements
if/then/else example
if today == “Monday” then
print(“Eugh! Monday again!”)
elseif today == “Tuesday” then
print(“Tuesday, one day closer the weekend!”)
elseif today == “Wednesday” then
print(“Half way there!”)
elseif today == “Thursday” then
print(“One more day to go!”)
elseif today == “Friday” then
print(“I can’t believe its Friday!”)
elseif today == “Saturday” then
print(“Woo! Its Saturday!”)
elseif today == “Sunday” then
print(“Aww, its Monday tomorrow!”)
else
print(“That’s not a day!”)
endif
switch/case statement example
switch entry:
case “Monday”:
print(“Eugh! Monday again!”)
case “Tuesday”
print(“Tuesday, one day closer the weekend!”)
case “Wednesday”:
print(“Half way there!”)
case “Thursday”:
print(“One more day to go!”)
case “Friday”:
print(“I can’t believe its Friday!”)
case “Saturday”:
print(“Woo! Its Saturday!”)
case “Sunday”:
print(“Aww, its Monday tomorrow!”)
default:
print(“That’s not a day!”)
endswitch
Iteration and selection statements can be nested, leading to a structure as shown below
Count ← 0
REPEAT
INPUT Score[Count]
IF Score[Count] >= 70 THEN
Grade[Count] ← "A"
ELSE
IF Score[Count] >= 60 THEN
Grade[Count] ← "B"
ELSE
IF Score[Count] >= 50 THEN
Grade[Count] ← "C"
ELSE
IF Score[Count] >= 40 THEN
Grade[Count] ← "D"
ELSE
IF Score[Count] >= 30 THEN
Grade[Count] ← "E"
ELSE
Grade[Count] ← "F"
ENDIF
ENDIF
ENDIF
ENDIF
ENDIF
Count ← Count + 1
UNTIL Count = 30
Each if statement contains another if statement which affects the flow of the program. This is however functionally identical to the days of the week program shown above
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?