Arrays (OCR A Level Computer Science)
Revision Note
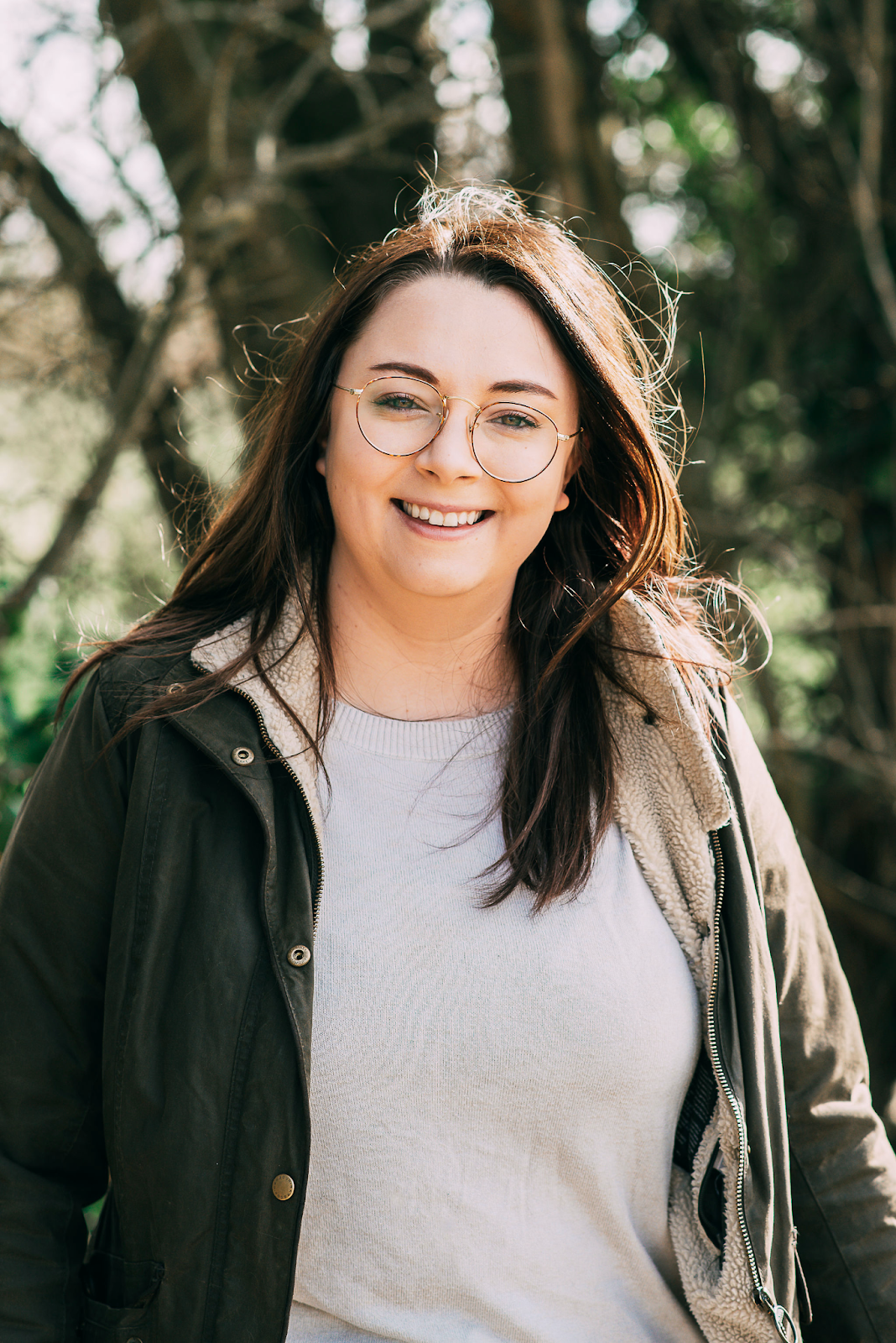
Author
Jennifer PageExpertise
Computer Science
1D Arrays
What is an Array?
An array is an ordered, static set of elements
Can only store 1 data type
A 1D array is a linear array
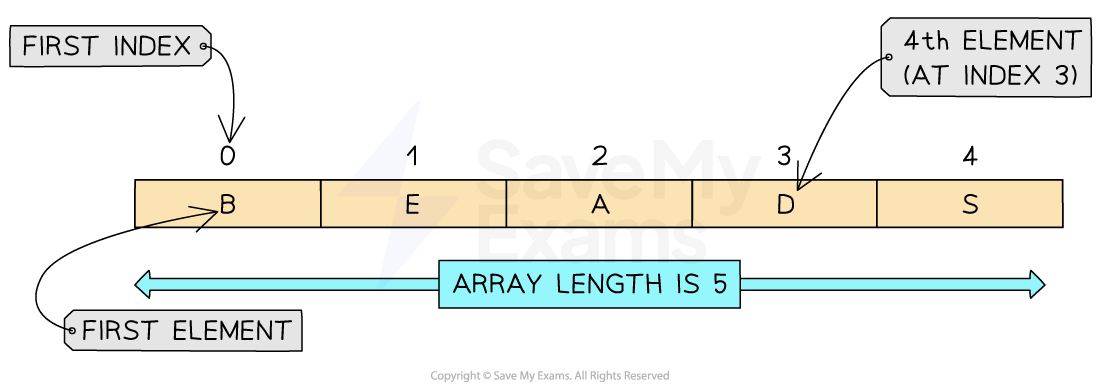
Structure of a 1D array
Example in Pseudocode
In this example we will be creating a one-dimensional array called ‘array’ which contains 5 integers.
To create the array we can use the following syntax:
array[0] = 1
array[1] = 2
array[2] = 3
array[3] = 4
array[4] = 5
We can access the individual elements of the array by using the following syntax:
array[index]
We can also modify the individual elements by assigning new values to specific indices using the following syntax:
array[index] = newValue
We can also use the len function to determine the length of the array by using the following syntax:
len(array)
In the example we have iterated through the array to output each element within the array. We have used a For Loop for this.
// Creating a one-dimensional array
array array[5]
array[0] = 1
array[1] = 2
array[2] = 3
array[3] = 4
array[4] = 5
// Accessing elements of the array
print(array[0])
print(array[2])
// Modifying elements of the array
array[1] = 10
print(array)
// Iterating over the array
for element in array
print(element)
// Length of array
length = len(array)
print(length)
Example in Python
Creating a one-dimensional array called ‘array’ which contains 5 integers.
Create the array with the following syntax:
array = [1, 2, 3, 4, 5]
Access the individual elements of the array by using the following syntax:
array[index]
Modify the individual elements by assigning new values to specific indices using the following syntax:
array[index] = newValue
Use the len function to determine the length of the array by using the following syntax:
len(array)
In the example the array has been iterated through to output each element within the array. A for loop has been used for this
# Creating a one-dimensional array
array = [1, 2, 3, 4, 5]
# Accessing elements of the array
print(array[0]) # Output: 1
print(array[2]) # Output: 3
# Modifying elements of the array
array[1] = 10
print(array) # Output: [1, 10, 3, 4, 5]
# Iterating over the array
for element in array:
print(element)
# Output:
# 1
# 10
# 3
# 4
# 5
# Length of the array
length = len(array)
print(length) # Output: 5
Example in Java
Creating a one-dimensional array called ‘array’ which contains 5 integers.
To create the array, use the following syntax:
int[] array = {1, 2, 3, 4, 5};
Access the individual elements of the array by using the following syntax:
array[index]
Modify the individual elements by assigning new values to specific indices using the following syntax:
array[index] = newValue;
Use the following syntax to print the array as a string:
arrays.toString(array)
Use the length function to determine the length of the array by using the following syntax:
array.length;
In the example, the array has been iterated through to output each element within the array. A for loop has been used for this
public class OneDimensionalArrayExample {
public static void main(String[] args) {
// Creating a one-dimensional array
int[] array = {1, 2, 3, 4, 5};
// Accessing elements of the array
System.out.println(array[0]); // Output: 1
System.out.println(array[2]); // Output: 3
// Modifying elements of the array
array[1] = 10;
System.out.println(Arrays.toString(array)); // Output: [1, 10, 3, 4, 5]
// Iterating over the array
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
// Output:
// 1
// 10
// 3
// 4
// 5
// Length of the array
int length = array.length;
System.out.println(length); // Output: 5
}
}
2D Arrays
A 2D array can be visualised as a table
When navigating through a 2D array you first have to go down the rows and then across the columns to find a position within the array
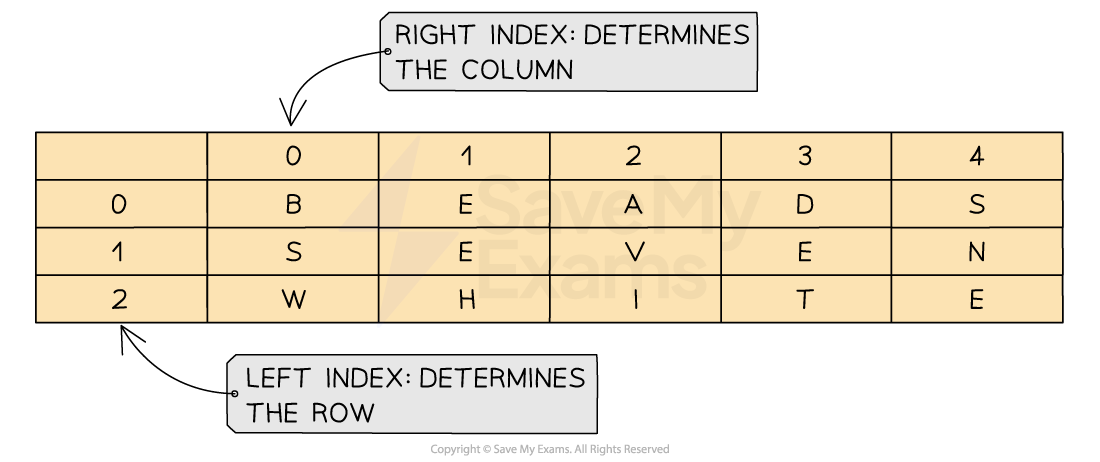
Structure of a 2D array
Example in Pseudocode
// Define the dimensions of the 2D array
ROWS = 3
COLS = 4
// Create a 2D array with the specified dimensions
array_2d = new Array[ROWS][COLS]
// Initialize the 2D array with values (optional)
for row = 0 to ROWS-1:
for col = 0 to COLS-1:
array_2d[row][col] = initial_value
// Accessing elements in the 2D array
value = array_2d[row_index][col_index]
Example in Python
# Method 1: Initialising an empty 2D array
rows = 3
cols = 4
array_2d = [[0 for _ in range(cols)] for _ in range(rows)]
# The above code creates a 2D array with 3 rows and 4 columns, filled with zeros.
# Method 2: Initialising a 2D array with values
array_2d = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# The above code creates a 2D array with 3 rows and 3 columns, with the specified values.
# Accessing elements in the 2D array
print(array_2d[0][0]) # Output: 1
print(array_2d[1][2]) # Output: 6
Example in Java
// Method 1: Initialising an empty 2D array
int rows = 3;
int cols = 4;
int[][] array2D = new int[rows][cols];
// The above code creates a 2D array with 3 rows and 4 columns, filled with zeros.
// Method 2: Initialising a 2D array with values
int[][] array2D = { {1, 2, 3},
{4, 5, 6},
{7, 8, 9} };
// The above code creates a 2D array with 3 rows and 3 columns, with the specified values.
// Accessing elements in the 2D array
System.out.println(array2D[0][0]); // Output: 1
System.out.println(array2D[1][2]); // Output: 6
3D Arrays
A 3D array can be visualised as a multi-page spreadsheet and can also be thought of as multiple 2D arrays
Selecting an element within a 3D array requires the following syntax to be used:
3DArrayName[z, y, x]
This is where z is the array index, y is the row index and x is the column index
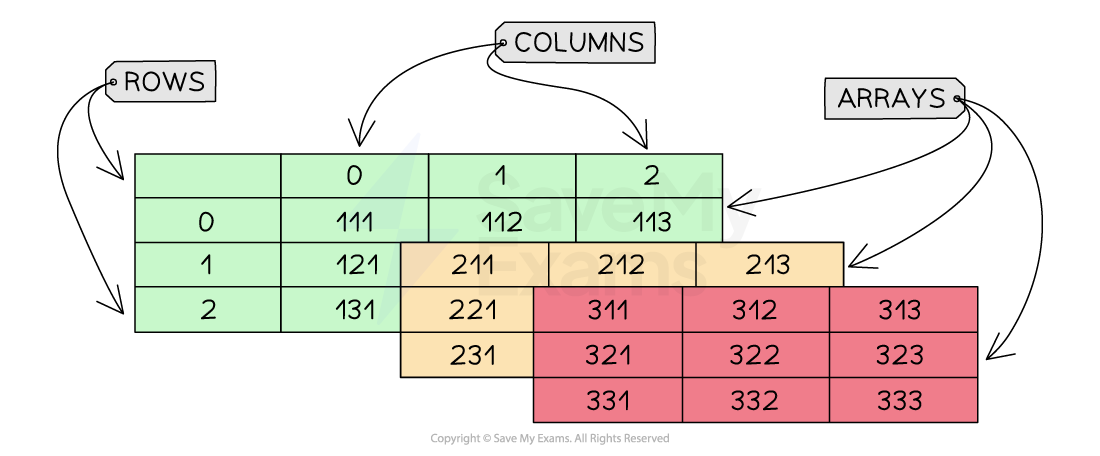
Structure of a 3D array
Example in Pseudocode
// Define the dimensions of the 3D array
ROWS = 3
COLS = 4
DEPTH = 2
// Create a 3D array with the specified dimensions
array_3d = new Array[ROWS][COLS][DEPTH]
// Initialize the 3D array with values (optional)
for row = 0 to ROWS-1:
for col = 0 to COLS-1:
for depth = 0 to DEPTH-1:
array_3d[row][col][depth] = initial_value
// Accessing elements in the 3D array
value = array_3d[row_index][col_index][depth_index]
Example in Python
# Method 1: Initialising an empty 3D array
rows = 3
cols = 4
depth = 2
array_3d = [[[0 for _ in range(depth)] for _ in range(cols)] for _ in range(rows)]
# The above code creates a 3D array with 3 rows, 4 columns, and 2 depths, filled with zeros.
# Method 2: Initialising a 3D array with values
array_3d = [[[1, 2], [3, 4], [5, 6], [7, 8]],
[[9, 10], [11, 12], [13, 14], [15, 16]],
[[17, 18], [19, 20], [21, 22], [23, 24]]]
# The above code creates a 3D array with the specified values.
# Accessing elements in the 3D array
print(array_3d[0][0][0]) # Output: 1
print(array_3d[1][2][1]) # Output: 14
Example in Java
// Method 1: Initialising an empty 3D array
int rows = 3;
int cols = 4;
int depth = 2;
int[][][] array3D = new int[rows][cols][depth];
// The above code creates a 3D array with 3 rows, 4 columns, and 2 depths, filled with zeros.
// Method 2: Initialising a 3D array with values
int[][][] array3D = { { {1, 2}, {3, 4}, {5, 6}, {7, 8} },
{ {9, 10}, {11, 12}, {13, 14}, {15, 16} },
{ {17, 18}, {19, 20}, {21, 22}, {23, 24} } };
// The above code creates a 3D array with the specified values.
// Accessing elements in the 3D array
System.out.println(array3D[0][0][0]); // Output: 1
System.out.println(array3D[1][2][1]); // Output: 14
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?