- Designing algorithms is a skill that must be developed and when designing algorithms, mistakes will be made
- There are two main types of errors that when designing algorithms a programmer must be able to identify & fix, they are:
- Syntax errors
- Logic errors
Types of Errors (AQA GCSE Computer Science)
Revision Note
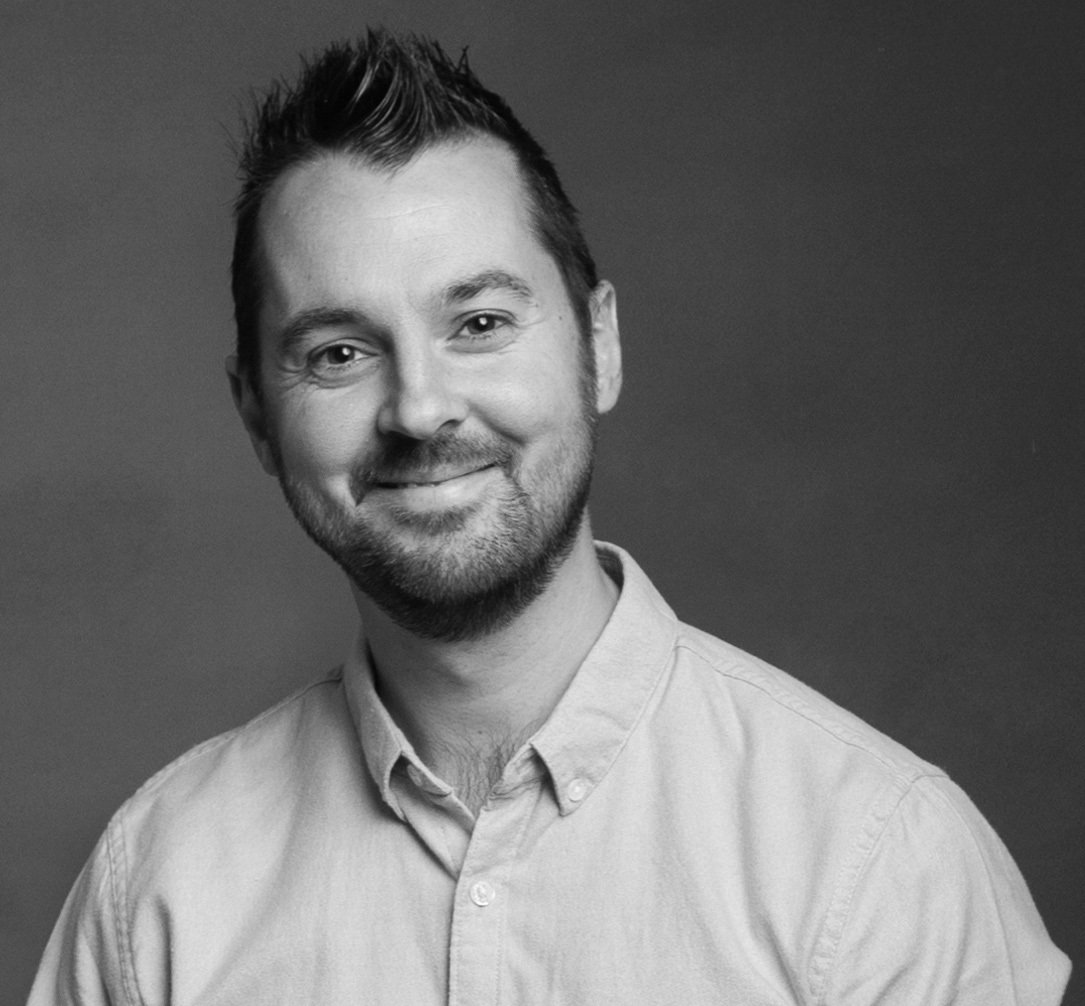
Author
James WoodhouseExpertise
Computer Science
Syntax & Logic Errors
What is a syntax error?
- A syntax error is an error that breaks the grammatical rules of a programming language and stops it from running
- Examples of syntax errors are:
- Typos and spelling errors
- Missing or extra brackets or quotes
- Misplaced or missing semicolons
- Invalid variable or function names
- Incorrect use of operators
- Incorrectly nested loops & blocks of code
Examples
Syntax Errors | Corrected |
|
|
|
|
What is a logic error?
- A logic error is where incorrect code is used that causes the program to run, but produces an incorrect output or result
- Logic errors can be difficult to identify by the person who wrote the program, so one method of finding them is to use 'Trace Tables'
- Examples of logic errors are:
- Incorrect use of operators (< and >)
- Logical operator confusion (AND for OR)
- Looping one extra time
- Indexing arrays incorrectly (arrays indexing starts from 0)
- Using variables before they are assigned
- Infinite loops
Example
- An algorithm is written to take as input the number of miles travelled. The algorithm works out how much this will cost, with each mile costing £0.30 in petrol. If this is greater than £10.00 then it is reduced by 10%.
Logic errors | Corrected |
|
|
Commentary | |
|
Identify & Categorise Errors
How can you identify and categorise errors?
- As a result of a syntax error breaking the rules of the programming language, the program will not execute
- These are easily identifiable as the IDE will provide information about what the error is
- This is done to help the programmer be able to fix the issue
- To help practise this skill, a selection of program screenshots will be used
The errors
- In the code below, there is a program which allows the user to enter how many items they wish to add to a shopping list
- The code then allows the user to
- Enter their chosen number of items into a list
- Output the list to the screen
- The code contains 3 syntax errors, highlighted with blue boxes
Error 1
- Line 3: Missing a second bracket at the end of integer input
- The error message below is what the IDE provided to help the programmer identify the error
- A top tip when programming is to look at the line of code above the one given in the error message
- For example, this error message claims line 6 is the issue, however, the code above line 6 (line 3) is the line that contains the error
Error 2
- Line 6: Missing a colon at the end of a for loop, while loop or if statement
- As mentioned earlier, the error message identifies the line after the actual error
- The error is on line 6, however, the syntax error message identifies the line below it
- Always check the line above for any potential errors
Error 3
- Line 7: Using == which is used for a comparison instead of a single = to declare a variable
- This would return the same error as above, this time the user would more easily be able to identify the issue as it is on line 7
The fix
- Once all fixes are in place, the code should appear as follows and the program should execute as intended
Identifying logic errors
- Logic errors can be more challenging to locate compared to syntax errors
- This is because the program will still run but will not produce the expected output that the programmer intended
- The most obvious areas to check for logic errors are:
- Logical operators (<, >, ==, !=)
- Boolean operators (AND, OR, NOT)
- Division by 0
- To help demonstrate this skill, another snippet of program code is used
- In this example, the incorrect Boolean operator has been used, OR instead of AND
- The result means the else clause in the if statement would never be caught
- At first glance, entering normal test data such as 14, the program works as intended
- Entering erroneous data or boundary test data which is outside of the range would result in the error
- When entering the age of 21, it still outputs that the user is in secondary school
- By changing the OR to AND, this corrects the logic error
Worked example
Nine players take part in a competition, their scores are stored in an array. The array is named scores and the index represents the player
Array scores
Index | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
Score | 7 | 9 | 2 | 11 | 8 | 4 | 13 | 10 | 5 |
The following program counts the total score of all the players
for x = 1 to 8
total = 0
total = total + scores[x]
next x
print(total)
When tested, the program is found to contain two logic errors.
Describe how the program can be refined to remove these logic errors [2]
How to answer this question
- A common logic error if an algorithm contains a loop is checking it loops the correct amount of times, how many times should this algorithm loop?
Answer
- For loop changed to include 0
- total = 0 moved to before loop starts
Guidance
- Moving total outside the loop is not enough, it could be moved after the loop which would still be a logic error)
- Corrected code accepted
total = 0
for x = 0 to 8
total = total + scores[x]
next x
print(total)
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?