- Taking an algorithm and turning it into code, in any language, requires an understanding of several basic programming concepts such as:
- Variables
- Constants
- Assignment
- Operators
- Inputs
- Outputs
Fundamental Programming Concepts (OCR GCSE Computer Science)
Revision Note
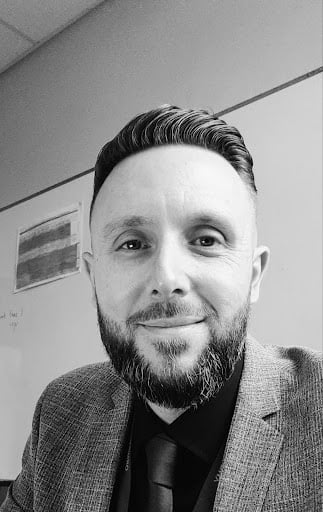
Author
Robert HamptonExpertise
Computer Science Content Creator
Variables, Constants & Assignments
What is a variable?
- A variable is a named memory location that holds data that during the execution of a program, the data can change
- Variables can store a variety of different types of data such as numbers, text or true/false values
- To store data in a variable, the process of assignment is used
What is a constant?
- A constant is fixed data that during the execution of a program cannot change
- A constant can store a variety of different types of data, similar to variables
- Pi is an example of a mathematical fixed value that would typically be stored as a constant
What is assignment?
- Assignment is the process of storing data in a variable or constant under a descriptive name
- Assignment is performed using the '=' symbol
Assigning variables & constants
Concept | OCR exam reference | Python |
Variables |
|
|
Constants |
|
|
Operators, Inputs & Outputs
What is an operator?
- An operator is a symbol used to instruct a computer to perform a specific operation on one or more values
- Examples of common operators include:
- Arithmetic
- Comparison
- Boolean (AND, OR and NOT)
Arithmetic
Operator | OCR exam reference | Python |
Addition | + |
+ |
Subtraction | - |
- |
Multiplication | * |
* |
Division | / |
/ |
Modulus (remainder after division) | MOD |
% |
Quotient (whole number division) | DIV |
// |
Exponentiation (to the power of) | ^ |
** |
Comparison
Operator | OCR exam reference | Python |
Equal to | == |
== |
Not equal to | != |
!= |
Less than | < |
< |
Less than or equal to | <= |
<= |
Greater than | > |
> |
Greater than or equal to | >= |
>= |
Examples
Operator | OCR exam reference | Python |
Addition | sum = 2 + 2 # 4 |
sum = 2 + 2 # 4 |
Multiplication | sum = 3 * 4 # 12 |
sum = 3 * 4 # 12 |
Modulus | sum = 10 MOD 3 # 1 |
sum = 10 % 3 # 1 |
Quotient | sum = 10 DIV 3 # 3 |
sum = 10 // 3 # 3 |
Exponentiation | sum = 2 ^ 2 # 4 |
sum = 2 ** 2 # 4 |
Equal to | if 3 == 3 then # True |
if 3 == 3: # True |
Not equal to | if 5 != 6 then # True |
if 5 != 6: # True |
Greater than or equal to | if 10 >= 2 then # True |
if 10 >= 2: # True |
AND |
|
|
What is an input?
- An input is a value that is read from an input device and then processed by a computer program
- Typical input devices include:
- Keyboards - Typing text
- Mice - Selecting item, clicking buttons
- Sensors - Reading data from sensors such as temperature, pressure or motion
- Microphone - Capturing audio, speech recognition
- Without inputs, programs are not useful as they can't interact with the outside world and always produce the same result
What is an output?
- An output is a value sent to an output device from a computer program
- Typical output devices include:
- Monitor - Displaying text, images or graphics
- Speaker - Playing audio
- Printer - Creating physical copies of documents or images
Area of a rectangle program
OCR exam reference |
|
Python |
|
Worked example
A cinema calculates ticket prices based on age category
- Adult = £13.00
- Child = £7.50
The program asks the user to enter their age and calculates the cost of their ticket
A simple algorithm is used
adult = 13.00
child = 7.50
age = input("What is your age: ")
if age > 18 then
total_cost = adult
else
toal_cost = child
end if
print(total_cost)
The cinema decides to add a discount of 25% to customers who come to the cinema on 'Sunday evening'
Identify all the additional inputs that will be required for this change to the algorithm [2]
How to answer this question
- What new information is needed?
Answer
- day
- time
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?