Programming Using Pre-Existing Libraries (Edexcel GCSE Computer Science)
Revision Note
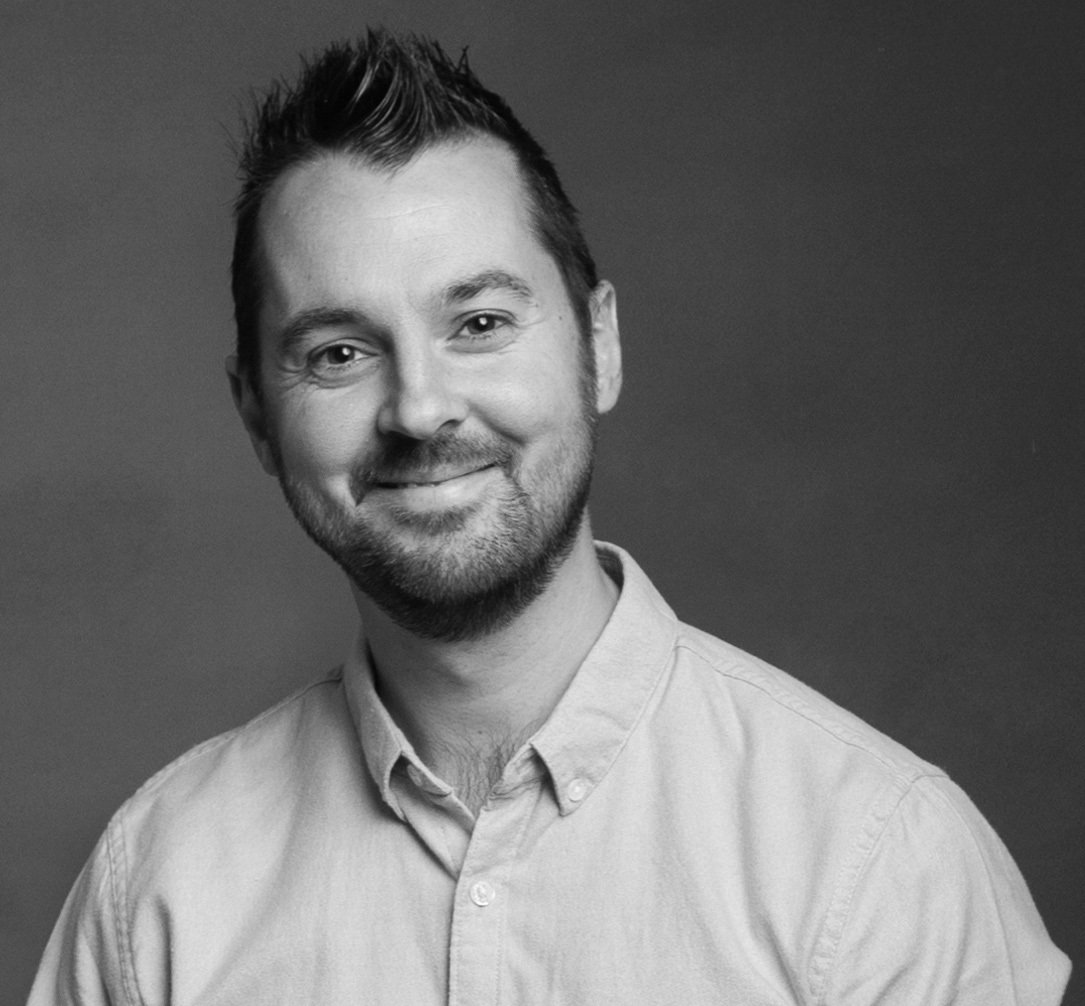
Author
James WoodhouseExpertise
Computer Science
Programming Using Pre-Existing Libraries
What is a pre-existing library?
A pre-existing library is reusable code that can be used throughout a program
The code has been made public through reusable modules or functions
Using pre-existing libraries saves programmers time by using working code that has already been tested
Some examples of pre-existing libraries are:
Random
Math
Turtle
Programming Random Library
What is the random library?
The random library is a library of code which allows users to make use of 'random' in their programs
Examples of random that are most common are:
Random choices
Random numbers
Random number generation is a programming concept that involves a computer generating a random number to be used within a program to add an element of unpredictability
Examples of where this concept could be used include:
Simulating the roll of a dice
Selecting a random question (from a numbered list)
National lottery
Cryptography
Concept | Python |
---|---|
Random numbers |
|
Random choice |
|
Examples in Python
Random code |
---|
# importing random library
# asking user to enter a username and password
# checking if the user and password are correct
|
National lottery |
---|
# Create a list of numbers for the national lottery
# Create an empty list to store the chosen numbers
# Loop to pick 6 numbers from the list
# Sort the chosen numbers in ascending order
# Output the chosen numbers
|
Programming Math Library
What is the math library?
The math library is a library of code which allows users to make use of mathematical operations which can be performed with ease
Examples of math that are most common are:
math.pi - the constant Pi
math.sqrt (x) - returns the square root of a number
math.floor(x) - returns the largest number, not less than x
math.ceil (x) - returns the smallest number, not less than x
Concept | Python | Output |
---|---|---|
Math.pi |
| 153.94 |
Math.sqrt(x) |
| 14.14 |
Math.floor(x) |
| 32 |
Math.ceil(x) |
| 33 |
Programming Turtle Library
What is the turtle library?
The turtle library is a pre-built library of code which allows the creation of images and shapes using a 'pen'
Examples of turtle that are most common include:
Polygons
Complex shapes such as spirographs
The turtle library uses a canvas which is based on X, Y coordinates
Turtle includes a series of simple instructions which can be used along with fundamental programming constructs to create drawings
It is important to note that just like all libraries, the user must import turtle at the beginning of the program
Concept | What it does | Python Example |
---|---|---|
turtle.pendown() | Puts the pen down ready to begin drawing |
|
turtle.penup() | Lifts the pen up to stop drawing if moving the pen |
|
turtle.forward(x) | Moves the turtle forward the number steps in the brackets |
|
turtle.right(x) | Turns the turtle right to the number of degrees specified |
|
turtle.left(x) | Turns the turtle left to the number of degrees specified |
|
turtle.setpos(x,y) | Moves the turtle to the position declared |
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?